Testing the architecture of your distributed .NET Aspire solution
Last year, I read a book called Fundamentals of Software Architecture: An Engineering Approach by Mark Richards and Neal Ford. The book comprehensively covered different architecture patterns, architecture characteristics, soft skills, and practices overall. One very interesting chapter delved into architectural testing, often referred to as fitness functions. Currently, I'm reading Software Architecture: The Hard Parts, which actually also touches on this architecture testing topic.
In this blog post, we will dive into architecture tests and handle the following questions: What exactly are architecture tests? Why should we write architecture tests? What tooling/libraries should we use to write architecture tests? Why is this topic important, especially now for .NET Aspire solutions in a monorepo?
Now, this topic of automated architecture tests is very topical in one work project because we're transforming one of our biggest applications into a monorepo and transforming the solution to the .NET Aspire. You can read more about that journey from my Finland Azure User Group presentation.
What are Architecture Tests / Fitness Functions?
Why are Architecture Tests needed?
👉 Architecture tests serve as a vital safeguard, ensuring the consistency of the solution architecture. Architecture tests make sure that design principles are followed.
👉 Enforce architecture conventions for class design, naming, and dependencies.
👉 Reduce the risk of human error during code reviews.
👉 Architecture tests are essential in monorepos because it's easy to violate the design principles in one shared codebase.
👉 Prevents architecture and design principle violations in the early phase.
👉 Architecture tests function similarly to unit tests. You can execute these tests in the CI/CD pipeline.
Tooling
The Fundamentals of Software Architecture book mentions a library called NetArchTests which enables a fluent way to create automated architecture tests in .NET environments. I'm using that library in the samples in this blog post.
GitHub page of NetArchTests says that: "The project allows you to create tests that enforce conventions for class design, naming, and dependency in .NET code bases. These can be used with any unit test framework and incorporated into a build pipeline. It uses a fluid API that allows you to string together readable rules that can be used in test assertions."
In this blog post, I'll handle mostly architecture tests from the dependency point of view.
Why are architecture tests important for distributed .NET Aspire solutions developed in a monorepo?
The .NET Aspire development model effectively encourages a monorepo approach. Monorepos improve the developer experience by keeping all project components close together, which enhances development, debugging, and testing efficiency. But all of this comes with great responsibility. A monorepo offers much freedom and flexibility, but it's easy to adopt practices that go against the architecture. Code reviews are essential for detecting architectural violations, but there is always a possibility of human error. Automated architectural tests play an essential role in identifying problems in an early phase.
This doesn't mean that you should use automated architecture tests only in a monorepo. Architecture tests are crucial for the success of every solution. It's also good to remember that a monorepo is not the only possibility for a distributed .NET Aspire application. You can keep every .NET Aspire microservice solution in its own repositories if you like and deploy everything in a shared Azure Container Apps Environment. Read more about this possibility from my previous blog post regarding .NET Aspire and multi-tenant platforms.
Sample distributed .NET Aspire solution in a monorepo
This sample distributed solution comprises two distinct microservices along with a centralized service defaults project that supports all microservices. Every microservice adheres to the principles of clean architecture. The dependency rule introduced by Uncle Bob (Robert C. Martin) is a fundamental principle to follow in clean architecture. The guideline states that dependencies should exclusively point inward. Practically, this means that the inner circle doesn't know anything about the outer circle.
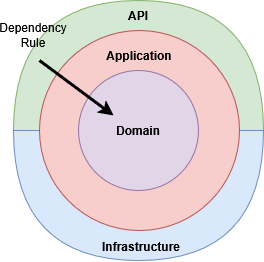
Foundation of each microservice
- Domain is the center and innermost element in your application. It's independent and has no reference to other layers. The domain contains business logic and domain entities.
- Application orchestrates the application's business logic and use cases.
- Infrastructure contains logic for handling communication to external systems, databases, etc.
- API is the outermost thin layer that represents the presentation layer in this solution.
Shared cross-cutting concerns
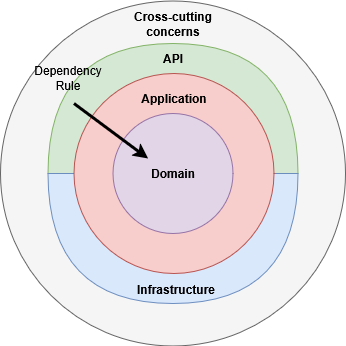
Shared cross-cutting concerns are located in the .NET Aspire Service Defaults project, which supports all microservices. The Service Defaults project is automatically generated by the .NET Aspire Visual Studio template and includes features for service discovery, health checks, resiliency, and logging. These capabilities are not business domain-specific.
Ideal state of the .NET Aspire solution
Each microservice is independent and strictly follows the clean architecture design principles.
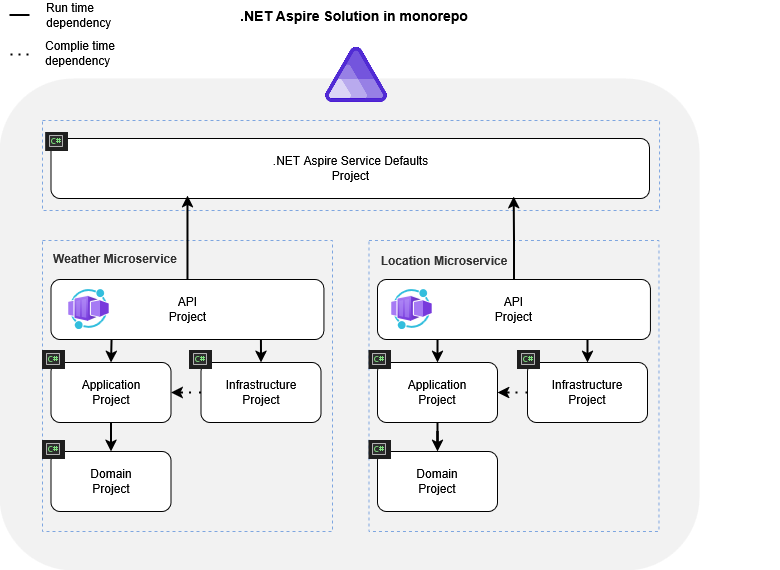
Dependency violations in monorepos
It's easy to compromise architecture in a monorepo solution, particularly regarding dependencies. Architecture tests ensure a consistent, clean architecture and prevent direct dependencies between microservices. The API Gateway will orchestrate calls between microservices (not shown in the diagram below).
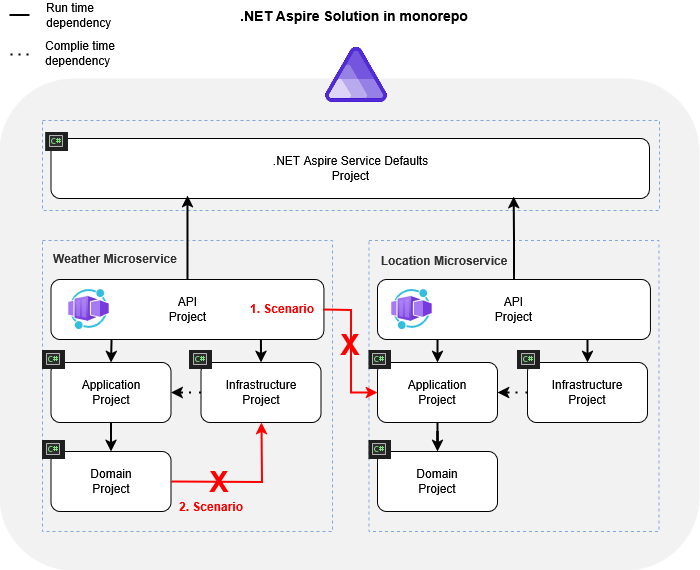
- Scenario: A direct dependency to another microservice component. Microservices should be independent and not rely on other microservice components directly.
- Scenario: A microservice has a dependency rule violation where the domain layer relies on infrastructure, which should be avoided to maintain independence and cleanliness in the architecture.
Next, let's deep dive into architecture tests and how to prevent these kinds of violations in practice using NetArchTest.
How to use NetArchTest to write architecture tests?
NetArchTest facilitates the creation of thorough architectural tests that validate dependencies, naming conventions, and more. In this sample, we will focus on testing those two scenarios introduced above.
Create a new xUnit unit test project specific to the microservice in the solution.
dotnet new xunit --name Aspire.FitnessFunctions.Location.Tests
Install NetArchTests and Shouldly Nuget-packages. I use Shouldly as an assertion framework in these samples.
Install-Package NetArchTests
Install-Package Shouldly
Locate all necessary assemblies of the solution for test project dependencies.
protected static readonly Assembly DomainAssembly = typeof(Location.Domain.Location).Assembly;
protected static readonly Assembly ApplicationAssembly = typeof(Location.Application.ILocationDbContext).Assembly;
protected static readonly Assembly InfrastructureAssembly = typeof(Location.Infrastructure.LocationDbContext).Assembly;
protected static readonly Assembly ApiAssembly = typeof(Location.Api.Endpoints.EndpointRouteBuilderExtensions).Assembly;
protected static readonly Assembly ServiceDefaultsAssembly = typeof(ServiceDefaults.Extensions).Assembly;
Create a first test to verify that the Location domain has no direct dependencies on the Weather domain components (1. Scenario violations).
[Fact]
public void LocationMicroservice_Should_NotHaveDependencyOnWeatherMicroservice()
{
var assemblies = new List<Assembly>
{
DomainAssembly,
ApplicationAssembly,
InfrastructureAssembly,
ApiAssembly
};
IEnumerable<Assembly> assemblyList = assemblies;
var result = Types.InAssemblies(assemblyList)
.That()
.ResideInNamespace("Aspire.FitnessFunctions.Location")
.ShouldNot()
.HaveDependencyOn("Aspire.FitnessFunctions.Weather")
.GetResult();
result.IsSuccessful.ShouldBeTrue();
}
Create a second test to verify that clean architecture principles are followed. Domain layer should not have a dependency on infrastructure (2. Scenario violations).
[Fact]
public void DomainLayer_Should_NotHaveDependencyOnInfrastructure()
{
var assemblies = new List<Assembly>
{
DomainAssembly,
ApplicationAssembly
};
IEnumerable<Assembly> assemblyList = assemblies;
var result = Types.InAssemblies(assemblyList)
.ShouldNot()
.HaveDependencyOn(
"Aspire.FitnessFunctions.Location.Infrastructure")
.GetResult();
result.IsSuccessful.ShouldBeTrue();
}
Summary
As we saw, automated architecture tests are a powerful way to enforce architecture and design principles. Architecture tests are an investment in the sustainability of your application.
Overall, NetArchTest is a comprehensive .NET library for creating architecture tests. The Fluent API approach of NetArchTest enables an easy, flexible, and intuitive way of creating architecture tests. I recommend reading through the NetArchTest documentation to get a full picture of its capabilities. Overall, it's great, and I'll definitely use it later in my projects.
Comments